File Manager > Extending Functionality
Customize File Filters
Learn how to add, replace, or remove filters in the File Manager.
This feature is available since Webiny v5.37.0.
- how to add a custom filter to the File Manager
- how to discover existing filter names
- how to change the position of filters, remove, or replace an existing filter
Overview
By default, File Manager offers a single built-in filter, to help you quickly filter files based on their type (images, documents, videos). To customize filters, you need to use the FileManagerViewConfig
component. For this article, we will use the Filter
component, located in the Browser
namespace.
Browser is the main area of the File Manager, where users browse files, apply filters, perform searching, organize files into folders, upload new files, etc.
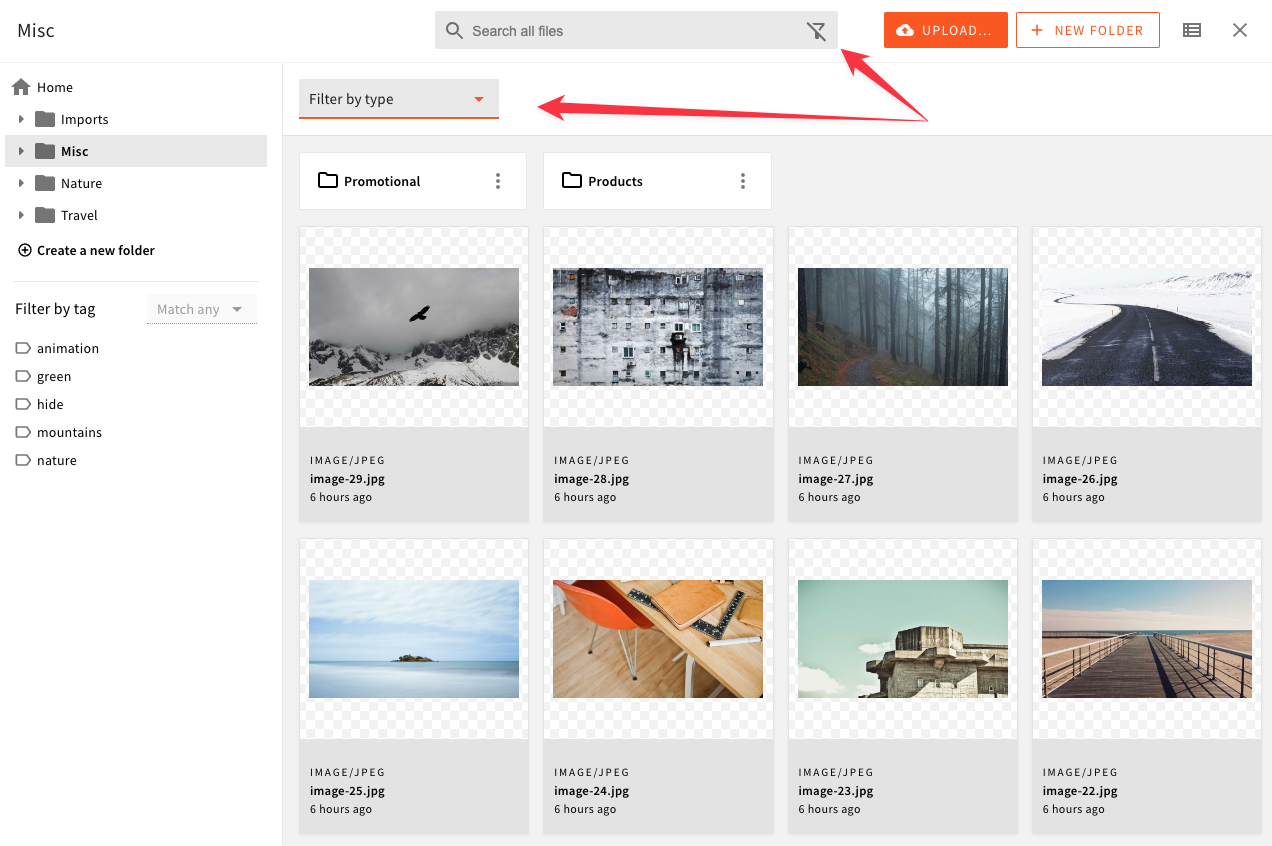
Add a Filter
To add a new filter, you need to use the FileManagerViewConfig
component and mount it within your Admin
app. Your filter is just a regular React element, so it can render anything.
Next time you open your File Manager, and expand the filters bar, you’ll see your new element there. This is the whole process of registering a new filter element. In the following sections, we’ll focus on implementing actual filtering.
Simple Filter
The following example shows how to implement a filter that toggles between “all files” and “large files”. For this example, we treat all files larger than 200KB as “large files”. Note that we are only showing the actual filter implementation. To see the filter registration process, revisit the previous section.
Under the hood, filters are handled via a regular form component. This means that we can simply hook into the form to set new filter values. For that, we’re using the useBind
hook, and give it a name. This name
will become a key within the form data object, which will in turn be passed to the GraphQL query. To unset a filter, we simply set undefined
. On button click, we toggle between the 2 values, and also show a label that corresponds to the current state of the filter.
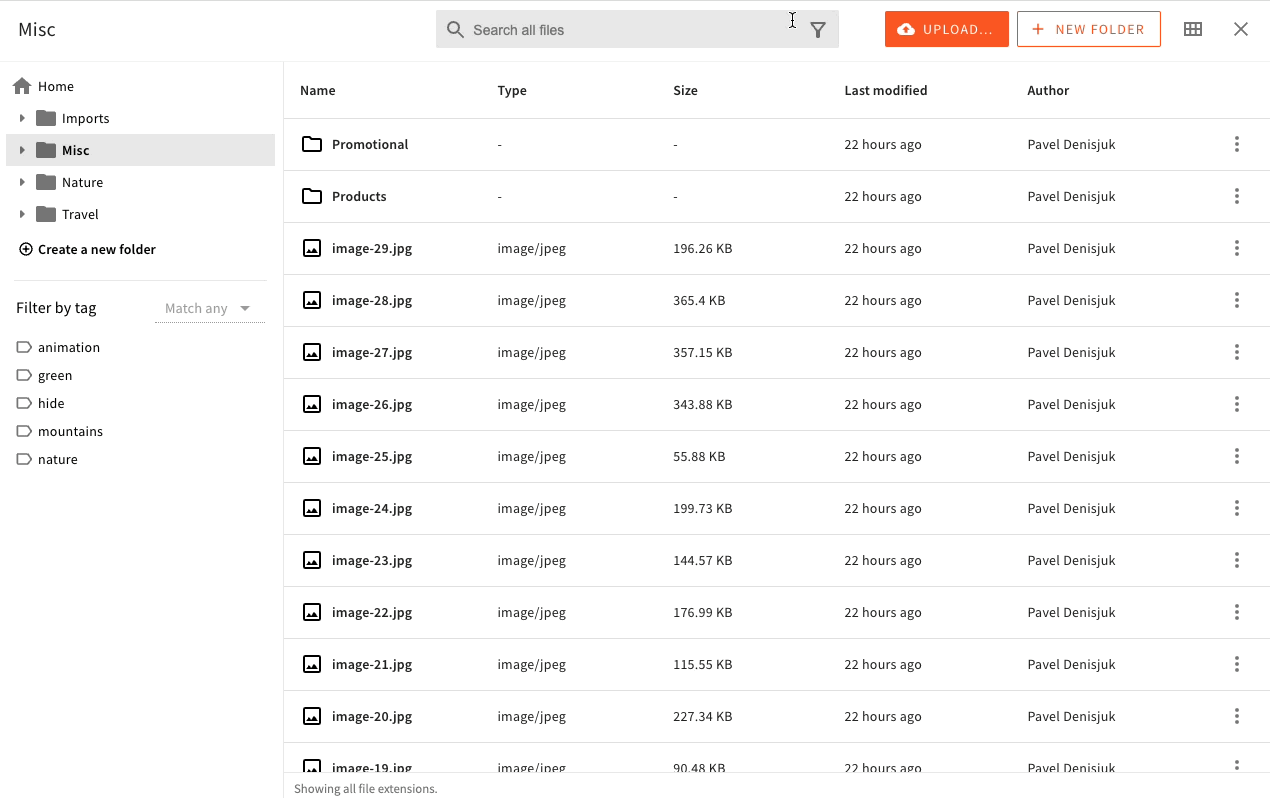
Advanced Filter
Sometimes, you need to implement filtering logic that is a bit more complex than just setting a single value. The following example shows a filter in form of a dropdown menu, where each option needs to use a different GraphQL query operator. > 200KB
needs to use the size_gt
key, while < 200KB
needs to use the size_lt
key. This cannot be achieved with simple form binding.
To correctly format our filters, we’ll use the Browser.FiltersToWhere
component to register our custom converter, which will allow you to convert form data to a valid GraphQL query input. With this approach, you have full control over values that will be passed to the API, when the user interacts with the filters UI.
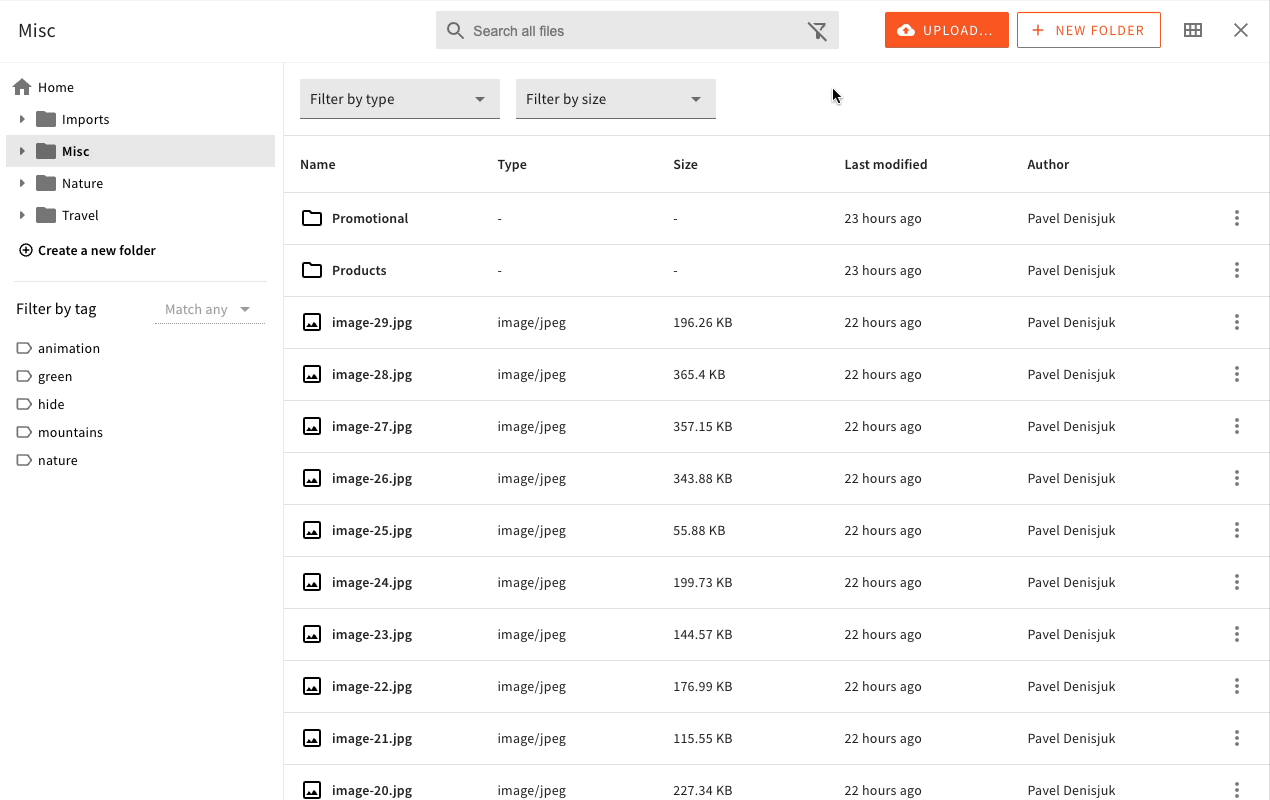
Discover Filters
This section demonstrates how you can discover the names of existing filters. This is important for further sections on positioning, removing, and replacing filters.
The easiest way to discover existing filters is to use the React Dev Tools plugins for your browser, and look for the Filters
element. From there, you can either look for filters
props, or look at the child elements and their keys:
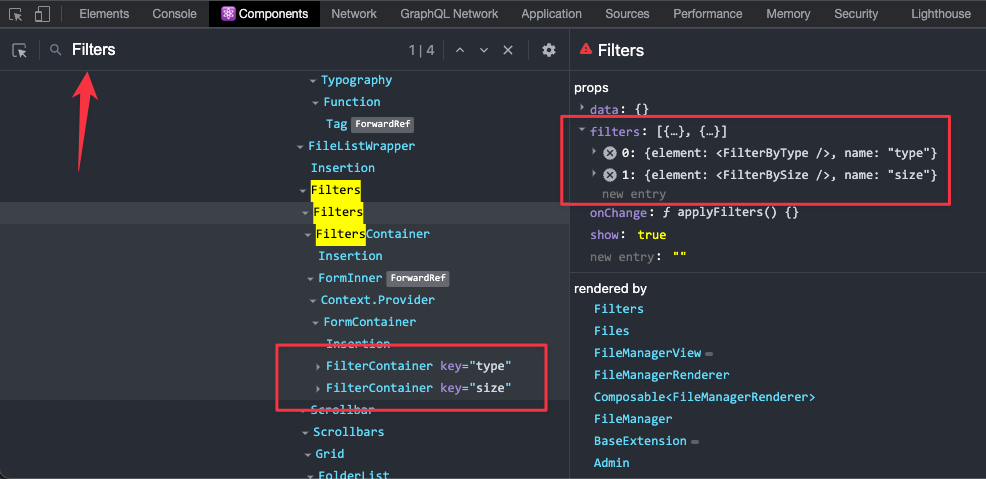
Position a Filter
To position your custom filter before or after an existing filter, you can use the before
and after
props on the <Filter>
element:
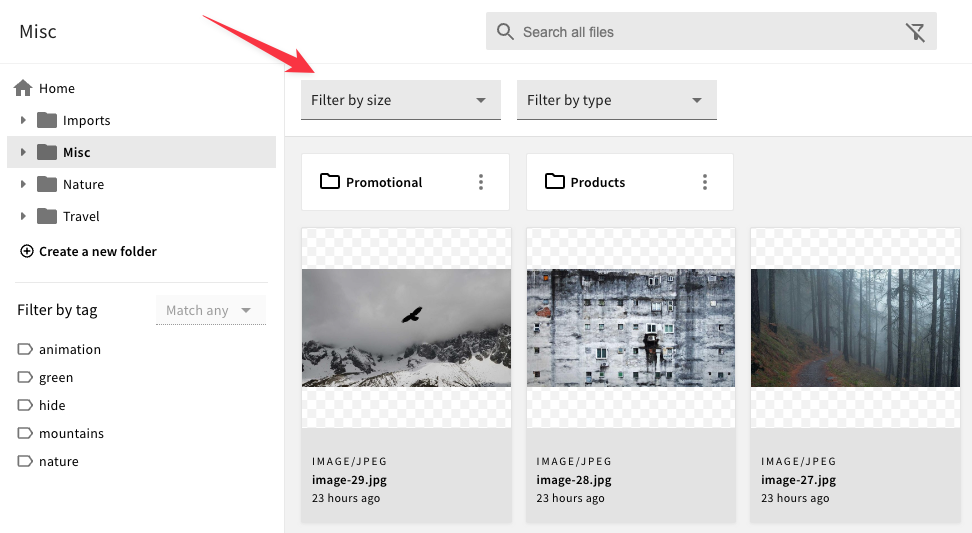
Remove a Filter
Sometimes you might want to remove an existing filter. All you need to do is reference the filter by name, and pass a remove
prop to the <Filter>
element:
Replace a Filter
To replace an existing filter with a new filter element, you need to reference the existing filter by name, and pass a new element via the element
prop: